9 FPGA Projects Ideas for Different Skill Levels
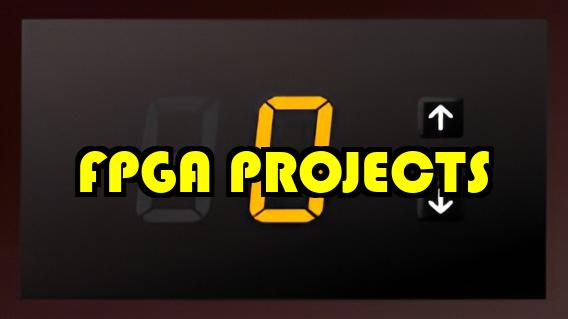
Basic LED Blinking:
A classic beginner project to get familiar with the FPGA development flow. Blink LEDs in various patterns and speeds using Verilog/VHDL.
Blinking an LED on an FPGA Project is a fantastic first project to understand the development flow and logic programming. Here’s a breakdown of how to achieve it:
Hardware:
- FPGA Development Board: Choose a board suitable for beginners, like Terasic DE1-SoC, Nexys 4, or Digilent Spartan-6 LX9. These boards have LEDs integrated or accessible through expansion headers.
- Programming Cable: A JTAG cable to connect your computer to the FPGA board for programming.
- Programming Software: Download the specific Integrated Development Environment (IDE) for your board, like Xilinx Vivado or Intel Quartus Prime.
Software:
- Verilog or VHDL: Choose your preferred Hardware Description Language (HDL) and learn its basic syntax for logic gates and registers.
Steps:
-
- Design the LED Circuit: Connect the LED with an appropriate current-limiting resistor to an output pin of your FPGA through a breadboard or dedicated board connectors. Refer to the board’s user manual for pin assignments and voltage specifications.
- Write the HDL Code:
-
- Clock Signal: Utilize the provided onboard clock signal (e.g., 100 MHz) as a reference for timing.
- Counter: Implement a counter within your code using registers.
- Blinking Logic: Use the counter value to control the LED state. Popular options include:
- Simple Toggle: Toggle the LED state (on/off) when the counter reaches a specific value and resets.
- Different Speeds: Vary the blinking rate by changing the reset value of the counter.
- Patterns: Create more complex patterns by involving multiple LEDs and toggling them based on different counter values or additional logic gates.
- Constrain the Design: Define pin mappings in a constraints file, linking your HDL signals to specific physical pins on the FPGA projects.
- Synthesize and Program: Use the IDE to synthesize your HDL code into a bitstream configuration file and program it onto the FPGA projects.
- Test and Debug: Observe the LED behavior, and if it isn’t blinking as expected, check your code and connections for errors. Use the debugger built into your IDE to identify and fix any issues.
Additional Tips:
- Start with a simple code like a single LED toggle and gradually add complexity.
- Refer to online tutorials and example FPGA Projects for specific boards and languages.
- Utilize the documentation and support resources provided by your FPGA vendor and community forums.
- Don’t hesitate to experiment and explore different blinking patterns and logic configurations.
Remember, blinking an LED is just the beginning! This FPGA Project helps you build a solid foundation for more advanced FPGA projects involving complex digital circuits and real-world applications.
ALSO READ : Power Rush Generator: Overview and Expert Guide On Working 2024
Have fun creating your blinking LED masterpiece!
7-Segment Display Counter:
Display numbers 0-9 on a 7-segment display, counting up or down at user-controlled speeds. Introduces basic counting logic and I/O interfacing.
Here’s a guide to make this FPGA project::
Hardware:
- FPGA Development Board: As mentioned earlier, choose a beginner-friendly board with 7-segment displays integrated or accessible through headers.
- 7-Segment Display(s): If not on-board, acquire single or multiple 7-segment displays (common anode or common cathode).
- Resistor(s): Each segment usually requires a current-limiting resistor (values vary based on LED type and supply voltage).
Software:
- Verilog or VHDL: Continue using your preferred HDL for this FPGA Project.
Steps:
- Design the Display Circuit:
-
-
- Common Anode vs. Common Cathode: Understand the type of your display and connect accordingly.
- Multiplexing (if needed): If using multiple displays, implement multiplexing to control them individually.
-
- Write the HDL Code:
-
- Counter Module: Create a counter module that increments/decrements based on user input (up/down buttons).
- Encoder Module: Design a module that converts the counter value (0-9) into 7-segment display patterns (segment activation signals).
- Multiplexing Logic (if applicable): Add logic to control which display is active at any given time.
- Constraints: Assign the counter output, encoder output, and display control signals to the appropriate FPGA pins.
- Incorporate User Inputs: Add logic to read buttons or switches for up/down control and speed adjustment.
- Synthesize and Program: Follow the same process as with the LED FPGA Project.
- Test and Debug: Observe the display behavior and ensure correct counting and control. Use debugging tools if needed.
Additional Tips:
- Segment Patterns: Refer to tutorials or datasheets for accurate segment activation patterns for each digit.
- Debounce Inputs: Implement debouncing techniques for user inputs to prevent unintended triggering.
- Clock Dividers: Use clock dividers to adjust counting speed based on user input or preset values.
- Experiment with Features: Explore adding features like pausing, resetting, or displaying hexadecimal values.
Remember:
- Start simple and gradually add complexity.
- Utilize online resources, vendor documentation, and community forums for support.
- Embrace experimentation and creativity!
This FPGA Project effectively builds upon your FPGA skills and introduces essential concepts like counting logic, I/O interfacing, and user interaction. It paves the way for more advanced digital projects involving displays and user controls.
Sine Wave Generator FPGA Projects:
Generate a digital sine wave signal using trigonometric calculations in the FPGA. Output the signal to a DAC (digital-to-analog converter) to create an audible tone.
You can generate a digital sine wave on an FPGA using trigonometric calculations and send it to a DAC for an audible tone. Here’s a breakdown of the process:
1. Phase Accumulation:
- Start by representing the phase of the sine wave digitally. You can use a fixed-point or floating-point accumulator to increment the phase at a specific rate determined by the desired output frequency.
- Each clock cycle, increment the phase accumulator by a value proportional to the desired frequency divided by the FPGA clock frequency.
- You can control the output frequency by varying the increment value.
2. Sine Calculation:
- Once you have the current phase, you need to convert it to a sine value. There are a few options:
- Direct Table Lookup (LUT): Pre-generate an array of sine values (sine table) in memory and use the phase as an index to read the corresponding sine value. This is efficient but limits the resolution and output frequency.
- CORDIC Algorithm: This iterative algorithm uses simple shifts and adds to approximate sine and cosine values. It’s computationally efficient but might require more logic resources.
- Taylor Series Approximation: Expand the sine function into a Taylor series and compute the first few terms based on the phase. This offers a balance between accuracy and resource usage.
3. Digital-to-Analog Conversion (DAC):
- Output the calculated sine value to a DAC to convert it into an analog voltage representing the sine wave.
- Choose a DAC with appropriate resolution and output range to match your desired signal amplitude and desired tone quality.
Additional Considerations:
- Filtering: The digitally generated sine wave might have quantization noise and harmonics. To produce a clean tone, use an analog low-pass filter after the DAC to remove unwanted frequencies.
- Amplitude control: Implement digital gain control to adjust the output amplitude of the sine wave.
- Synchronization: If you need multiple tones or synchronized outputs, consider using additional clocking and phase alignment circuits.
Remember that the specific implementation of these steps will depend on your chosen FPGA platform, DAC requirements, and desired tone quality. If you share more details about your FPGA Project goals and the FPGA platform you’re using, I can provide more specific guidance and resources.
ALSO READ: GE Circuit Breakers: Best Guide On Powering a Safer Tomorrow
Audio Player with SD Card:
Design an audio player that reads MP3 files from an SD card and outputs the audio through a speaker. Requires interfacing with external memory and audio codecs.
Designing an audio player with an SD card and audio codec on an FPGA is a challenging but rewarding FPGA Projects. Here’s a breakdown of the key steps involved:
1. System Architecture:
- MP3 Decoder: Choose an MP3 decoder IP core compatible with your FPGA platform. Popular options include the VS1053B and the DFPlayer Mini. These cores handle MP3 decoding and provide audio data and control signals.
- SD Card Interface: Implement an SD card interface using the FPGA’s built-in SD controller or an external SD card controller IP core. This interface allows reading and writing data to the SD card for accessing MP3 files.
- Audio Codec: Integrate an audio codec IC to convert the digital audio data from the MP3 decoder to an analog signal for driving a speaker. Choose a codec based on your desired audio quality and output power.
- Control Logic: Design the control logic using HDL (Hardware Description Language) like VHDL or Verilog to manage data flow, playback controls, and communication between the decoder, SD card interface, and audio codec.
2. MP3 Decoding:
- The MP3 decoder IP core typically provides an interface for configuring decoding parameters like bitrate and sampling rate.
- The decoder outputs the decoded audio data as PCM (Pulse Code Modulation) samples, usually in I2S format (Inter-IC Sound).
3. SD Card Interface:
- Implement the SD card interface protocol according to the chosen SD card controller. This involves managing clock signals, data transfer, and command sequences for reading MP3 files from the SD card.
- You can use libraries or IP cores provided by the FPGA vendor to simplify the SD card interface implementation.
4. Audio Codec Integration:
- Connect the I2S output of the MP3 decoder to the input of the audio codec IC.
- Configure the codec based on the desired audio format (e.g., sample rate, bit depth) and output driver characteristics for driving the speaker.
5. Control Logic and User Interface:
- Design the control logic to manage playback operations like play, pause, stop, and track selection. This can involve user input through buttons or an external interface like a serial port.
- Implement a display or indicator using LEDs or an LCD to show track information or playback status.
Additional Considerations:
- Power Supply: Ensure adequate and stable power supply for the FPGA, SD card, and audio codec.
- Audio Filtering: Implement analog low-pass filters after the audio codec to remove unwanted high-frequency noise.
- Volume Control: Include a digital or analog volume control circuit to adjust the output audio level.
Remember that the specific implementation details will depend on your chosen FPGA platform, MP3 decoder IP core, audio codec, and desired features. Consulting the datasheets and reference designs provided by the component manufacturers can be very helpful.
Image Processing Filter:
Implement a basic image filter (e.g., grayscale conversion, edge detection) on an incoming video stream using the FPGA’s parallel processing capabilities
Here’s a guide to make this FPGA project:
1. Choose your filter:
- Grayscale conversion: Converts color images to black and white by averaging the red, green, and blue channels.
- Edge detection: Identifies sharp changes in intensity, highlighting edges and object boundaries. Popular methods include Sobel and Canny filters.
2. Understand the video stream interface:
- Pixel Format: Determine the color space (RGB, YCbCr) and bit depth of each pixel.
- Data Streaming: Analyze the data format (parallel/serial) and clocking protocol used to transfer pixel data to the FPGA.
3. Design the filter pipeline:
- Line Buffer: Store incoming pixel data to create a window for applying the filter over contiguous pixels.
- Filter Core: Implement the chosen filter algorithm using logic blocks. This could involve arithmetic operations, comparisons, thresholding, etc.
- Output Processing: Prepare the filtered pixels for further processing or display, based on the intended output format.
4. Leverage FPGA benefits:
- Parallelism: Exploit the FPGA’s parallel architecture to process multiple pixels simultaneously, increasing performance.
- Pipelining: Implement pipelining within the filter core to overlap computation and data fetching, further optimizing throughput.
5. Tools and Resources:
- Hardware Description Language (HDL): Use VHDL or Verilog to describe the filter logic and control flow.
- IP Cores: Consider pre-built IP cores for common image processing functions like color space conversion or specific edge detection algorithms.
- Development Kits: FPGA development kits often provide reference designs and tools for video processing applications.
Additional Tips:
- Start with a simpler filter like grayscale conversion before exploring more complex algorithms.
- Use simulation tools to verify your design functionality before implementing it on the FPGA hardware.
- Consider optimizing your design for memory usage and resource utilization to fit within your specific FPGA model constraints.
Remember, this is a general guide, and the specific implementation details will depend on your chosen filter, video stream format, and available FPGA Project resources. Feel free to ask further questions for more specific guidance on your chosen filter or any aspect of the implementation process!
Game of Life:
Bring the famous cellular automata simulation to life on an FPGA! Display the evolving patterns on LEDs or a VGA screen.
Here’s a roadmap to bring the Game of Life to life on an FPGA:
1. Understand the rules:
- Each cell is alive or dead.
- A living cell with less than 2 or more than 3 live neighbors dies.
- A dead cell with exactly 3 live neighbors comes alive.
2. Design the grid:
- Choose a grid size (e.g., 16×16) that fits your FPGA Project resources and display capabilities.
- Represent each cell state (alive/dead) with a single bit in memory.
3. Parallel processing:
- Leverage the FPGA’s inherent parallelism to update every cell simultaneously.
- Design a logic circuit that takes each cell’s current state and the number of live neighbors to determine its next state.
4. Neighborhood calculation:
- Efficiently determine the number of live neighbors for each cell.
- Consider using dedicated logic blocks or shift registers to implement logic for checking surrounding cells.
5. Output and visualization:
- Choose your output method:
- LEDs: Directly map cell states to LEDs for a classic cellular automata display.
- VGA: Generate video signals to display the evolving patterns on a VGA monitor.
- Design logic to convert cell states into appropriate LED signals or VGA color values.
6. Optimization:
- Optimize your design for speed and resource utilization:
- Pipelining: Overlap computation and memory access to increase throughput.
- Look-up tables: Pre-calculate neighbor counts for specific cell configurations.
7. Tools and resources:
- Hardware Description Language (HDL): Use VHDL or Verilog to describe your logic circuits and control flow.
- Development Kits: Utilize FPGA development kits with available libraries and tools for video processing or LED control.
- Reference Designs: Search for existing Game of Life implementations on FPGAs for inspiration and optimization techniques.
Additional Tips:
- Start with a smaller grid size and test your design thoroughly before scaling up.
- Consider adding features like user input for setting initial patterns or controlling simulation speed.
- Experiment with different visualization techniques to create visually appealing displays of the evolving patterns.
This is a general guide for this FPGA project, and the specific implementation details will depend on your chosen grid size, output method, and desired features. Feel free to ask further questions for more specific guidance on your Game of Life FPGA Project!
PID Temperature Controller:
Design a closed-loop control system using an FPGA that reads temperature sensor data and adjusts heating/cooling elements to maintain a desired temperature. Here’s a roadmap to building a closed-loop temperature control system using an FPGA:
1. System Components:
- Temperature Sensor: Choose a sensor compatible with your FPGA and suitable for your desired temperature range (e.g., thermistor, thermocouple).
- Analog-to-Digital Converter (ADC): Convert the sensor’s analog voltage signal to a digital value the FPGA can process.
- Digital-to-Analog Converter (DAC): Convert the FPGA’s control signal back to an analog voltage to drive the heating/cooling element.
- Heating/Cooling Element: Select an actuator based on your application (e.g., Peltier cooler, heating resistor).
2. PID Control Algorithm:
- Implement the Proportional-Integral-Derivative (PID) algorithm in your FPGA using HDL (VHDL or Verilog).
- The PID controller takes the difference between the measured temperature and the desired setpoint as its input.
- Based on this error, it calculates an output control signal that adjusts the heating/cooling element to minimize the error over time.
3. Design Considerations:
- Sample Rate: Choose an appropriate sampling rate for the ADC to capture temperature changes accurately.
- PID Tuning: Adjust the PID gains (Proportional, Integral, and Derivative) for optimal performance and stability based on your system dynamics.
- Calibration: Account for sensor offset and gain errors to ensure accurate temperature measurement.
4. FPGA Implementation:
- Use dedicated logic blocks (LUTs, FFs) or soft-core processors for implementing the PID algorithm and control logic.
- Integrate the ADC and DAC interfaces using the FPGA’s built-in peripherals or external IP cores.
- Consider implementing safety features like over-temperature protection and alarm mechanisms.
5. Tools and Resources:
- Development Kit: Utilize an FPGA development kit with necessary peripherals and libraries for ADC/DAC communication.
- Simulation Tools: Simulate your design in HDL simulators to verify functionality before hardware implementation.
- PID Tuning Tools: Tools and software are available to assist with PID parameter tuning for optimal performance.
Visualization and Monitoring:
- Display the measured temperature, setpoint, and control signal on LEDs, LCDs, or through serial communication.
- Consider implementing data logging features to record and analyze system behavior over time.
Remember This is a general guide for this FPGA project, and the specific implementation details will depend on your chosen components, desired precision, and safety requirements. Feel free to ask further questions for more specific guidance or assistance with any aspect of your PID temperature controller FPGA Project!
Simple RISC-V Processor:
Another FPGA Project is a basic RISC-V processor core on the FPGA that can execute simple assembly language programs. Introduces advanced concepts like pipelining and instruction fetch/decode. Building a basic RISC-V processor core on an FPGA introduces fundamental concepts like instruction execution, pipelining, and memory access. Here’s a roadmap to get you started:
1. Choose a RISC-V ISA subset:
- Start with a small subset of the RISC-V instruction set architecture (ISA) like RV32I (32-bit integer instructions). This simplifies design complexity and focuses on core elements.
2. Design the processor core:
- Datapath: Define the processor’s functional units like ALU, registers, and control logic using hardware description language (HDL) like VHDL or Verilog.
- Register File: Implement a small register file (e.g., 8-16 registers) to store temporary data and program variables.
- ALU: Design the Arithmetic Logic Unit (ALU) to perform basic arithmetic and logical operations on data.
- Instruction Fetch/Decode: Implement logic to fetch instructions from memory, decode them into control signals, and access required operands.
3. Pipelining (Optional):
- Introduce pipelining to overlap instruction fetch, decode, and execution stages, improving performance. This requires careful synchronization and buffering of data between pipeline stages.
4. Memory Interface:
- Connect the processor to external memory (e.g., SDRAM) for storing instructions and data. Use appropriate protocols and address decoding logic to access memory locations.
5. Simulation and testing:
- Use HDL simulators to test and debug your processor design before implementing it on the FPGA hardware. Utilize test benches and assembly language programs to verify correct functionality.
6. Tools and Resources:
- FPGA Development Kit: Choose a development kit with sufficient logic resources and memory capacity for your processor design.
- RISC-V Toolchain: Use an open-source RISC-V toolchain (e.g., GNU Toolchain) to compile assembly language programs into machine code instructions.
- Reference Designs: Explore existing open-source RISC-V processor designs like PicoRV for inspiration and learning resources.
Advanced Options:
- Implement additional RISC-V instructions beyond the baseline RV32I subset.
- Integrate peripherals like timers, interrupt controllers, and communication interfaces.
- Explore more advanced pipelining techniques for further performance gains.
Remember This is a general guide for this FPGA project, and the specific implementation details will depend on your chosen RISC-V ISA subset, target FPGA platform, and desired features. Feel free to ask further questions for more specific guidance or assistance with any aspect of your simple RISC-V processor FPGA Project!
Accelerated Cryptography Engine:
Implement a hardware accelerator for a popular cryptographic algorithm (e.g., AES) to significantly improve encryption/decryption performance. Here’s a general guide for this FPGA project:
1. Algorithm Selection:
- Choose the cryptographic algorithm to accelerate. AES (Advanced Encryption Standard) is a common choice due to its widespread use and well-defined structure.
2. Algorithm Analysis:
- Thoroughly understand the algorithm’s operations and data flow.
- Identify computationally intensive components and potential bottlenecks.
- Analyze data dependencies and opportunities for parallelism.
3. FPGA Architecture:
- Select an FPGA with sufficient resources (logic cells, memory, DSP blocks) to accommodate the design.
- Consider hardware features that can enhance cryptographic performance, such as dedicated hardware multipliers or block RAMs.
4. Hardware Design:
- Datapath: Implement the algorithm’s core operations using FPGA logic blocks and hardware multipliers.
- Control Logic: Coordinate data movement, manage keys, and control the overall encryption/decryption process.
- Memory: Allocate memory blocks for storing keys, intermediate data, and final results.
- Interface: Design a communication interface to exchange data with external systems (e.g., processors, memory controllers).
5. Optimization:
- Pipelining: Overlap computation and data transfers to increase throughput.
- Unrolling: Replicate critical blocks to reduce loop overheads.
- Loop Optimization: Rearrange loop structures for better resource utilization.
- Resource Sharing: Explore sharing hardware elements for multi-functional operations.
6. Testing and Validation:
- Simulation: Use HDL simulators to verify functionality and identify design errors.
- Hardware Testing: Implement the design on the FPGA and test with various input data and key combinations.
- Performance Validation: Measure encryption/decryption speed and compare it to software implementations.
7. Additional Considerations:
- Security: Protect sensitive keys and data from potential attacks.
- Side-Channel Attacks: Implement countermeasures against power analysis or timing-based attacks.
- Power Consumption: Optimize design for low power consumption, especially for battery-powered or energy-efficient applications.
Tools and Resources:
- FPGA Development Kit
- Hardware Description Language (VHDL or Verilog)
- HDL Simulation Tools
- FPGA Synthesis and Implementation Tools
- AES Algorithm Specifications and Test Vectors
- Open-Source FPGA Cryptographic Libraries (optional)
Making a secure and efficient cryptography engine FPGA Project requires a solid understanding of cryptographic algorithms, FPGA architecture, and hardware design principles. Seek guidance and resources from FPGA vendors, online communities, and academic publications for comprehensive support.
Conclusion
These are just starting points! Feel free to adapt and expand these ideas based on your interests and skill level. There’s a whole world of possibilities waiting to be explored in the exciting realm of FPGA projects.